Basic Cookie Clicker Code
This code creates a simplified Cookie Clicker game with: ✅ A clickable cookie
✅ A counter to display the number of cookies
✅ An auto-clicker upgrade
1. HTML (index.html)
This sets up the game interface:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Cookie Clicker</title>
<style>
body {
text-align: center;
font-family: Arial, sans-serif;
background-color: #f5deb3;
}
#cookie {
width: 200px;
cursor: pointer;
transition: transform 0.1s;
}
#cookie:active {
transform: scale(0.9);
}
#stats {
font-size: 24px;
margin: 20px;
}
.upgrade {
background-color: #ffcc00;
border: none;
padding: 10px;
margin: 10px;
cursor: pointer;
font-size: 18px;
}
</style>
</head>
<body>
<h1>Cookie Clicker</h1>
<p id="stats">Cookies: <span id="cookieCount">0</span></p>
<img id="cookie" src="https://upload.wikimedia.org/wikipedia/commons/a/a6/Cookie_icon.png" alt="Cookie">
<div>
<button class="upgrade" onclick="buyUpgrade()">Buy Auto Clicker (+1 CPS) - Cost: <span id="upgradeCost">10</span></button>
</div>
<script src="script.js"></script>
</body>
</html>
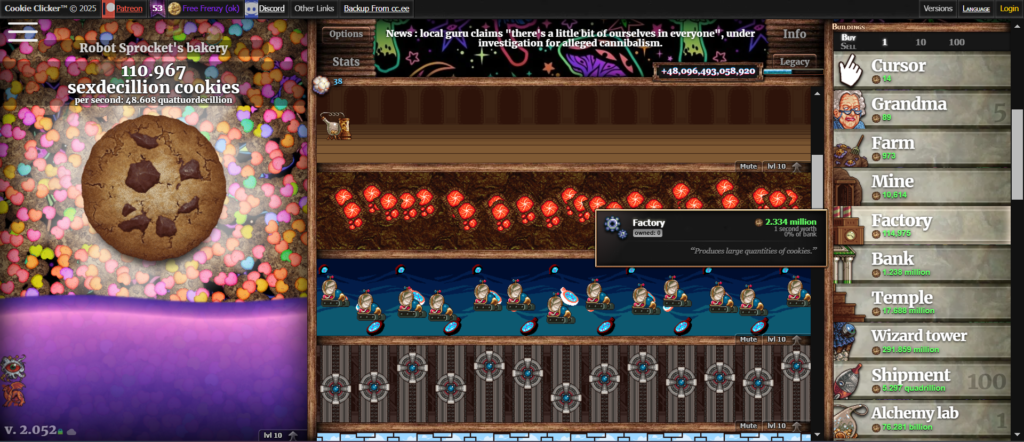
2. JavaScript (script.js)
This file controls the game mechanics:
// Game variables
let cookies = 0;
let cps = 0; // Cookies per second
let upgradeCost = 10; // Initial upgrade cost
// Update the displayed cookie count
function updateCookies() {
document.getElementById("cookieCount").innerText = cookies;
}
// Click event for the big cookie
document.getElementById("cookie").addEventListener("click", function() {
cookies++;
updateCookies();
});
// Function to buy an auto-clicker upgrade
function buyUpgrade() {
if (cookies >= upgradeCost) {
cookies -= upgradeCost;
cps++;
upgradeCost = Math.floor(upgradeCost * 1.5); // Increase cost by 50%
document.getElementById("upgradeCost").innerText = upgradeCost;
updateCookies();
} else {
alert("Not enough cookies!");
}
}
// Auto-generate cookies every second
setInterval(function() {
cookies += cps;
updateCookies();
}, 1000);
How This Code Works
- Click the Cookie:
- Clicking the cookie increases the cookie count by 1.
- Buy Upgrades:
- The auto-clicker costs 10 cookies initially and adds +1 CPS.
- The upgrade cost increases by 50% each time you buy it.
- Cookies Per Second (CPS):
- Every second, the game adds the CPS amount to the total cookies.
Expanding the Game
To make this more like the real Cookie Clicker, you could:
- Add more upgrades (e.g., Grandmas, Farms, Factories).
- Implement Golden Cookies that appear randomly.
- Use local storage to save progress when the player closes the page.
This is a basic Cookie Clicker clone in JavaScript! You can improve it further by adding more mechanics like the Grandmapocalypse, Synergies, and Heavenly Upgrades. 🍪🚀