1. Create an HTML File
Start by creating a file named index.html
. This file will define the structure of the game.
HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Cookie Clicker</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f0f0f0;
}
#cookie {
width: 200px;
cursor: pointer;
margin: 20px;
}
#stats {
font-size: 20px;
margin: 20px;
}
.upgrade {
margin: 10px;
padding: 10px;
background-color: #fff;
border: 1px solid #ccc;
border-radius: 5px;
cursor: pointer;
display: inline-block;
}
.upgrade:hover {
background-color: #f9f9f9;
}
</style>
</head>
<body>
<h1>Cookie Clicker</h1>
<p id="stats">Cookies: 0</p>
<img id="cookie" src="https://upload.wikimedia.org/wikipedia/commons/a/a6/Cookie_icon.png" alt="Cookie">
<div>
<button class="upgrade" onclick="buyUpgrade(1)">Buy Cursor (+1 CPS) - Cost: 10</button>
<button class="upgrade" onclick="buyUpgrade(2)">Buy Grandma (+5 CPS) - Cost: 50</button>
</div>
<script src="script.js"></script>
</body>
</html>
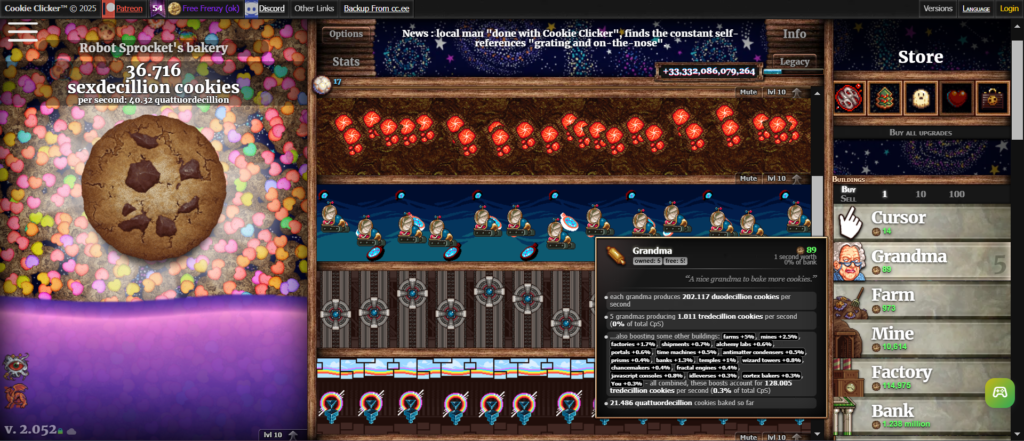
2. Add JavaScript Logic
Create a script.js
file in the same directory as your index.html
. This file will handle the game’s functionality, including cookie clicking, upgrading, and counting cookies per second.
JavaScript Code (script.js):
// Game Variables
let cookies = 0; // Total cookies
let cps = 0; // Cookies per second
let upgrades = [
{ cost: 10, cps: 1, amount: 0 }, // Cursor
{ cost: 50, cps: 5, amount: 0 } // Grandma
];
// Update the stats on the screen
function updateStats() {
document.getElementById("stats").innerText = `Cookies: ${Math.floor(cookies)} (CPS: ${cps})`;
}
// Handle cookie clicking
document.getElementById("cookie").addEventListener("click", function () {
cookies++;
updateStats();
});
// Buy an upgrade
function buyUpgrade(upgradeIndex) {
const upgrade = upgrades[upgradeIndex];
if (cookies >= upgrade.cost) {
cookies -= upgrade.cost;
upgrade.amount++;
cps += upgrade.cps;
upgrade.cost = Math.floor(upgrade.cost * 1.15); // Increase the cost by 15%
updateUpgrades();
updateStats();
} else {
alert("Not enough cookies!");
}
}
// Update upgrade buttons with new costs
function updateUpgrades() {
const buttons = document.querySelectorAll(".upgrade");
buttons[0].innerText = `Buy Cursor (+1 CPS) - Cost: ${upgrades[0].cost}`;
buttons[1].innerText = `Buy Grandma (+5 CPS) - Cost: ${upgrades[1].cost}`;
}
// Generate cookies per second
setInterval(function () {
cookies += cps / 10; // Generate cookies every 100ms
updateStats();
}, 100);
// Initialize
updateStats();
3. Save and Open the Game
- Save your files in the same folder:
index.html
script.js
- Open the
index.html
file in your browser (double-click it or open it via your browser’s File > Open menu).
4. How It Works
- Cookie Clicking: Clicking the cookie increases the total cookie count.
- Upgrades:
- The “Cursor” adds 1 Cookie Per Second (CPS) for each purchase.
- The “Grandma” adds 5 CPS for each purchase.
- The cost of upgrades increases by 15% with each purchase.
- Cookies Per Second: The game generates cookies automatically based on your total CPS.
5. Add Customizations (Optional)
Enhance the Game with These Features:
- Sound Effects: Add a click sound when the cookie is clicked.
- More Upgrades: Add more upgrade types, like Farms, Factories, etc.
- Save Progress: Use
localStorage
to save and load the game state. - Animations: Add CSS animations for clicking the cookie or purchasing upgrades.
- Golden Cookies: Introduce rare clickable bonuses for extra fun.
This setup creates a simple version of Cookie Clicker. You can expand on this framework to make it more engaging and advanced! 🍪